将一个类的接口转换成客户希望的另外一个接口。使得原本由于接口不兼容而不能一起工作的那些类可以一起工作。
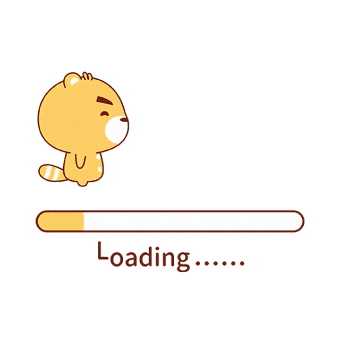
优点:
(1) 将目标类和适配者类解耦,通过引入一个适配器类来重用现有的适配者类,无须修改原有结构。
类图
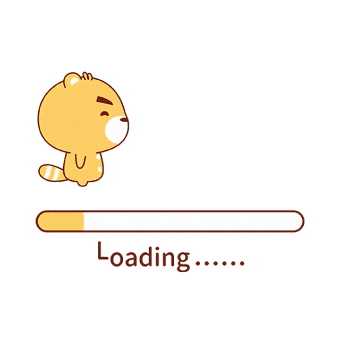
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| package main
import "fmt"
type V5 interface { UseV5() }
type V220 struct{}
func (v *V220) User220v() { fmt.Println("使用220V的电压") }
type Adapter struct { V220 *V220 }
func (a *Adapter) UseV5() { a.V220.User220v() fmt.Println("使用适配器进行220V到5V") }
func NewAdapter(v220 *V220) *Adapter { return &Adapter{v220} }
type Phone struct { v V5 }
func (p *Phone) charge() { fmt.Println("chargin 5v") p.v.UseV5()
} func NewPhone(v V5) *Phone { return &Phone{v} }
func main() { adapter := NewAdapter(new(V220)) phone := NewPhone(adapter) phone.charge() }
|
练习
适配下面的代码,目的是盖伦使用技能电脑就关机。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| package main
import "fmt"
type Attack interface { Fight() }
type ESkill struct{}
func (e *ESkill) Fight() { fmt.Println("使用e技能将敌人击杀") }
type Hero struct { Name string Attack Attack }
func (h *Hero) Skill() { fmt.Println("使用了技能") h.Attack.Fight() }
type PowerOff struct{}
func (p *PowerOff) ShutDown() { fmt.Println("电脑即将关机。。。") } func main() { gl := Hero{"盖伦", new(ESkill)} gl.Skill() }
|
修改后的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| package main
import "fmt"
type Attack interface { Fight() }
type ESkill struct{}
func (e *ESkill) Fight() { fmt.Println("使用e技能将敌人击杀") }
type Hero struct { Name string Attack Attack }
func (h *Hero) Skill() { fmt.Println("使用了技能") h.Attack.Fight() }
type PowerOff struct{}
func (p *PowerOff) ShutDown() { fmt.Println("电脑即将关机。。。") }
type Adapter struct { PowerOff *PowerOff }
func (a *Adapter) Fight() { a.PowerOff.ShutDown() } func NewAdapter(PowerOff *PowerOff) *Adapter { return &Adapter{PowerOff} } func main() { a := NewAdapter(new(PowerOff)) gl := Hero{"盖伦", a} gl.Skill() }
|